OK, this is the thread I said I was going to post in the "Revised Networking Paradigm" thread.
Part of this concept is stuff that I came up with last year when brain-storming ideas for the FPM tree. I wanted to have priests be able to use some skill that would create a magical beacon that would have a visual effect and remain in place for some number of seconds, adding an positive effect (Game::Effect) to surrounding friendly units and/or a negative effect to surrounding enemy units. This "beacon of hope" (or some such) shouldn't occupy any cells. There is currently no paradigm in place for such an object. AttackParticleSystems also do not occupy cells, but they wont fit the bill.
What is needed is an entirely new paradigm. When on my plane trip, I analyzed this as well and here is roughly what I came up with.
Last year, when enhancing particle systems, I added the class Shared::Graphics::Entity (functionally, an interface) that Game::Unit's now derive from. This gave me the ability to specify Units in an abstract fashion, in code in the shared_lib without needing to know the details of the "Entity" (specifically, so that an AttackParticleSystem could have an explicit target that it tracked and never missed).
namespace Shared { namespace Graphics {
class Entity {
public:
virtual ~Entity(){}
virtual Vec2i getPos() const = 0;
// virtual int getSize() const = 0;
virtual Vec3f getCurrVector() const = 0;
virtual Vec3f getCurrVectorFlat() const = 0;
};
}} // end namespace
However, this is insufficient now. We need a much more generic "game object" and I propose that the name "Entity" is perfect for this (I did a Quake mod a long time ago, so I came to like this term). Probably, "GameObject" would work equally well and I'm not hard-set on the name. Either way, I have a basic break-down, but I'm not certain what OO mechanisms to use for each (i.e., composition, vs inheritance, etc.). This is a VERY immature model!
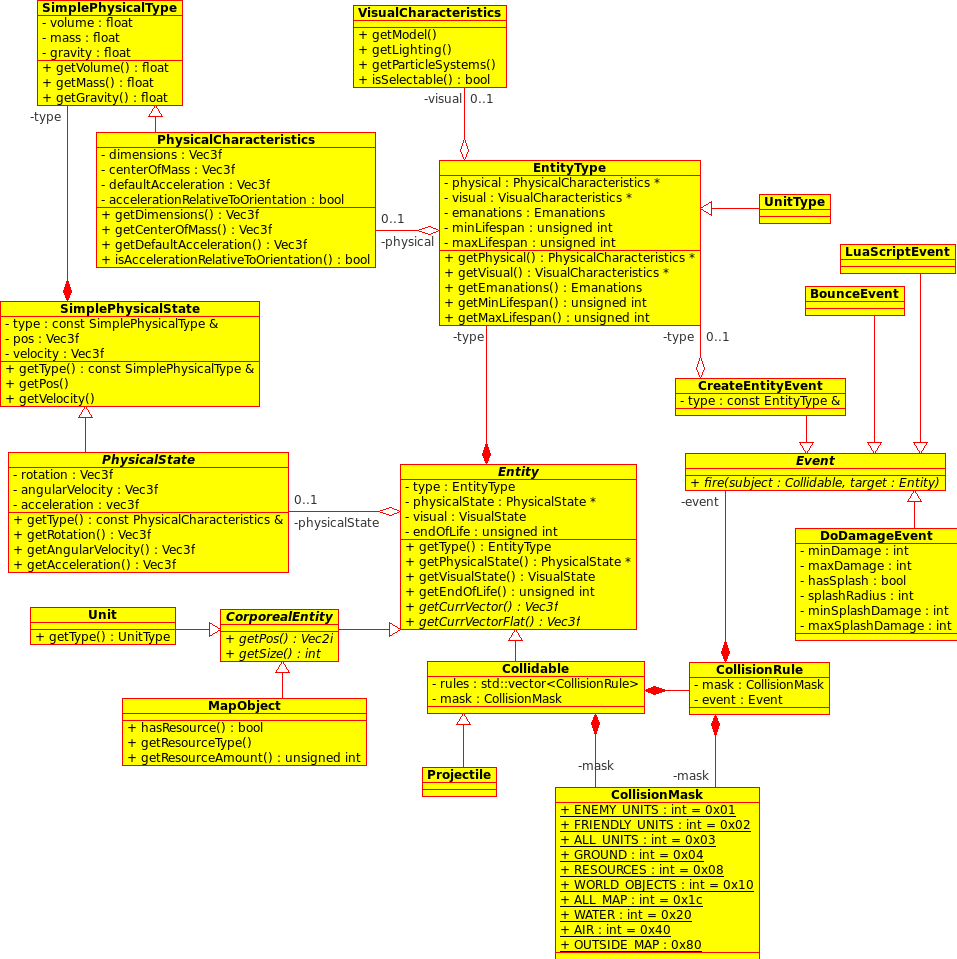
I really haven't sorted out who should inherit from what. For instance, the
VisualCharacteristics I would normally consider a "type" class, but for Units, the model changes depending upon what action they are taking, so just having a straight
getModel() or even
getAnimation() just wont work for
Units, where as it will work for projectiles (think of the arrows that archers shoot).
Emanations (see
source/game/types/effect_type.h:188) do seem to fit quite nicely into an abstract
EntityType class however.
PhysicalCharacteristics is one of those things that may or may not be present. I'm not sure if we should use a pointer (or tr1::shared_ptr) or a sub-class. The problem with using inheritance for stuff like this is that, inevitably, something in the scheme of what you're doing will change and you find that the type hierarchy you chose for the original problem no longer matches. That's why I'm leaning more towards using pointers and having this "type" information optional. For instance, I want projectiles to be able to be run through ODE (physics engine) for which, I will need
PhysicalCharacteristics and a
PhysicalState. The same is true for the advanced death sequences we worked on last year.
A
CorporealEntity is a specialization of an Entity that lives in cells and can thus have a Vec2i position that specifies an x, y coordinate on the map. Again, I'm not cetain of this precise class design. All entities will have (what is now called) a currVector, which is their location in 3D space according to OpenGL's coordinate system.
Perhaps
Collidable is unnecessary as a class. Perhaps what is needed instead is just it's fields & getter methods copied into either
Entity or
EntityType. This is what will give a generic object the ability to cause damage as AttackParticleSystems currently do. But the particle system(s) will be maintained separately, generating particles based upon their specification. Thus, there will be no more "splash particle systems" and "attack particle systems". These will be mere visual artefacts of a more flexible design. (My design above doesn't support for visuals related to impact, but it can be added to the Event class). Note that I'm saying ParticleSystem
s (plural). I've concluded that the path to really cool visual effects is allowing the definition of compound particle systems, each of which may have completely different definitions. I figure we should also be able to spawn objects with Events that will, in turn, have their own particle systems and exist for no other purpose than visual effect (this is where impact with the world can be handy, to terminate the visual effect).
For "tossable" objects, discussed last year with in the
GAE 0.3 planning thread under "Improved Death Sequences", collision events can be used for bouncing. This design also paves the way for Glestimals (discussed in that thread as well).
Finally, if an Entity isSelectable(), then a getDesc() method will have to be implemented.
In summary, these concepts are quite a long way from complete, but this is progress and these design changes will pave the way for making the game immensely more flexible -- once we sort out how to do it correctly.

EDIT: There's a few things here that aren't obvious:
- PhysicalCharacteristics' basicDimensions field is an optional field that can be used to give the engine a cheaper way to determine collisions and wind drag, an alternative to using the mesh from the model. This way, you can define alternate dimensions for these, even though you already have a model.
- Some CollisionMask values may not be intuitive.
- AIR is any time altitude is >= 0 and WATER is anytime it is <= 0.
- OUTSIDE_MAP is what happens when you travel outside of the area defined by the map (where units can't go) -- it's good to have objects terminate themselves there so they don't use up resources unnecessarily.
- Hitting a map resource will trigger both RESOURCE and WORLD_OBJECT, since resources are both, but hitting a rock or statue (for instance) will only trigger WORLD_OBJECT.
[/list]